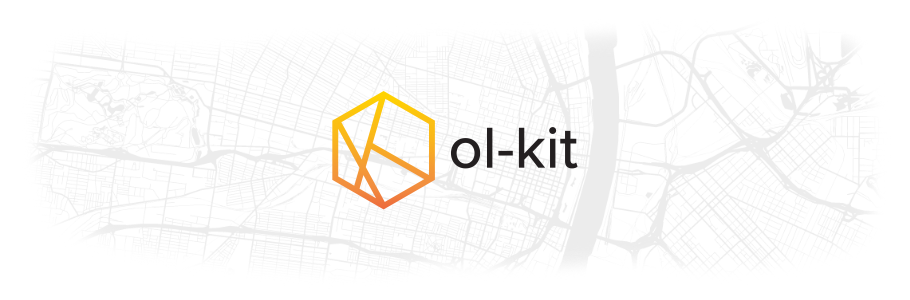
An easy to use, open source React & OpenLayers map component toolkit.
Checkout the demo site here!
Prebuilt Map Components
Ready to use and just work with the map via a single line drop-in!
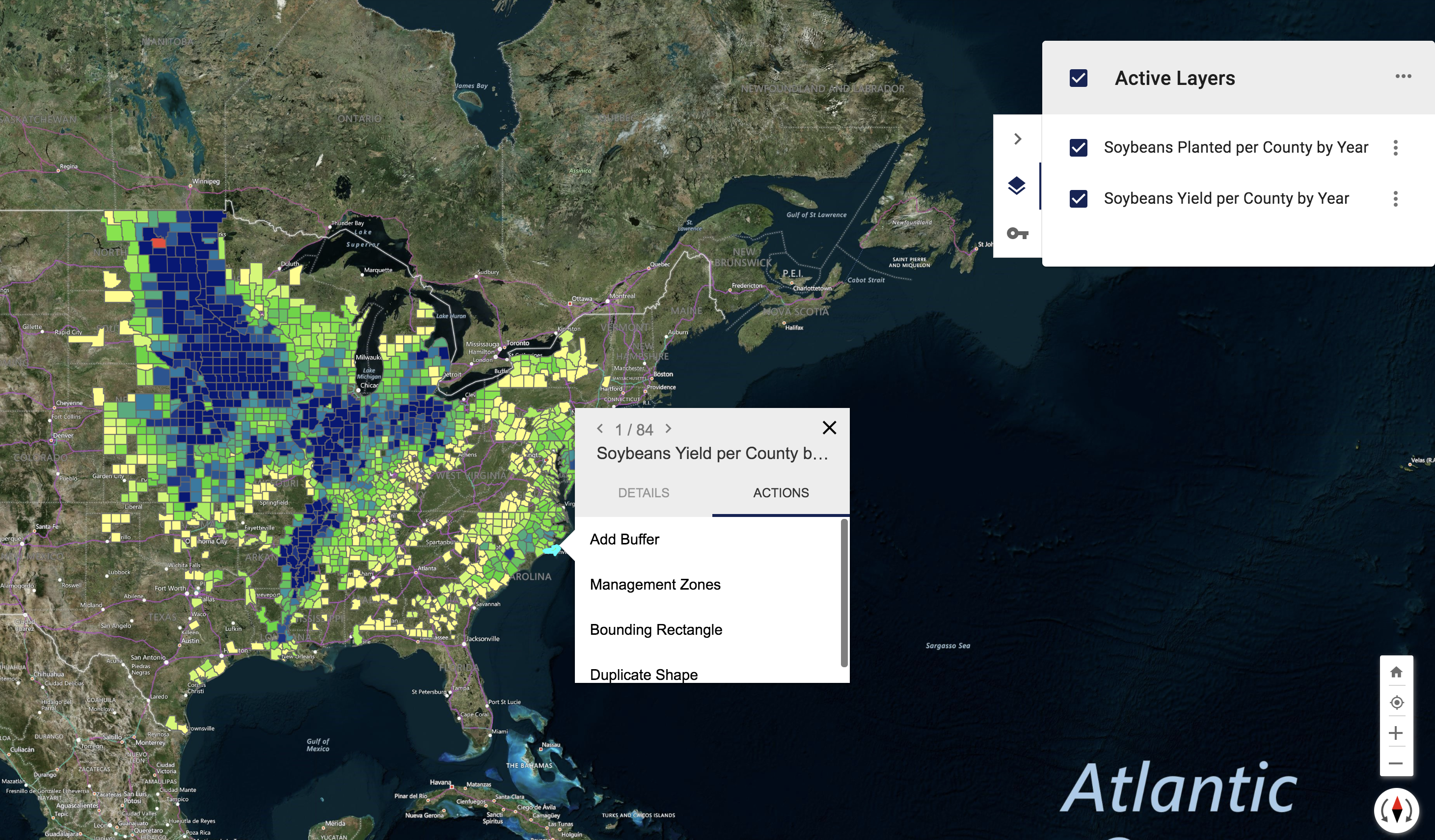
Installation
Install ol-kit and its peerDependencies
npm i @bayer/ol-kit ol react react-dom styled-components @material-ui/core @material-ui/icons @material-ui/styles --save
Getting Started
It's easy to start building map apps with ol-kit. For simple projects the following will get you started:
import React from 'react'
import {
Map,
BasemapContainer,
ContextMenu,
Controls,
LayerPanel,
Popup,
loadDataLayer
} from '@bayer/ol-kit'
class App extends React.Component {
onMapInit = async map => {
console.log('we got a map!', map)
// nice to have map set on the window while debugging
window.map = map
// find a geojson or kml dataset (url or file) to load on the map
const data = 'https://data.nasa.gov/api/geospatial/7zbq-j77a?method=export&format=KML'
const dataLayer = await loadDataLayer(map, data)
// set the title on the layer to show in LayerPanel
dataLayer.set('title', 'NASA Data')
console.log('data layer:', dataLayer)
}
render () {
return (
<Map onMapInit={this.onMapInit} fullScreen>
<BasemapContainer />
<ContextMenu />
<Controls />
<LayerPanel />
<Popup />
</Map>
)
}
}
export default App